public class JTableAdd_03 extends JFrame implements ActionListener{
Container cp;
JTextField tfSang,tfSu,tfDan;
JButton btnAdd,btnDel;
JLabel lbl1,lbl2,lbl3;
DefaultTableModel model;
JTable table;
int selectRow=-1;
//파일명
static final String FILENAME="/Library/sist0730/tdata.txt";
public JTableAdd_03(String title) {
super(title);
cp=this.getContentPane();
cp.setBackground(new Color(171,190,190));
this.setBounds(700,100,600,600);
this.initDesign();
//테이블 생성후 파일추가
tableReadData();
//종료
this.addWindowListener(new WindowAdapter() {
@Override
public void windowClosing(WindowEvent e) {
//데이터 저장
tableDataSave();
System.exit(0);
}
});
this.setVisible(true);
}
//종료시 저장할 메서드
//저장 바나나:3:1000:3000.. 마지막 콜론은 없애기
public void tableDataSave() {
FileWriter fw=null;
try {
fw=new FileWriter(FILENAME);
for(int i=0;i<table.getRowCount();i++) //getRowCount 행개수
{
String s=""; //string 초기화
for(int j=0;j<table.getColumnCount();j++) //getColumnCount dufrotn
{
s+=table.getValueAt(i, j)+":";
}
//마지막 한글자 : 제거
s=s.substring(0,s.length()-1);
fw.write(s+"\n");
}
} catch (IOException e) {
} finally {
try {
fw.close();
} catch (IOException e) {
}
}
}
//처음 생성시 테이블에 불러올 메서드
public void tableReadData() {
FileReader fr=null;
BufferedReader br=null;
try {
fr=new FileReader(FILENAME);
br=new BufferedReader(fr);
while(true)
{
String s=br.readLine();
if(s==null)
break;
String[]data=s.split(":");
//테이블에 배열형 데이터 추가
model.addRow(data);
}
} catch (FileNotFoundException e) {
} catch (IOException e) {
} finally {
try {
if(br!=null) br.close();
if(fr!=null) fr.close();
} catch (IOException e) {
}
}
}
public void initDesign() {
this.setLayout(null);
JLabel lbl1=new JLabel("상품명");
JLabel lbl2=new JLabel("수량");
JLabel lbl3=new JLabel("단가");
lbl1.setBounds(30, 20, 80, 30);
this.add(lbl1);
lbl2.setBounds(230, 20, 80, 30);
this.add(lbl2);
lbl3.setBounds(430, 20, 80, 30);
this.add(lbl3);
tfSang=new JTextField();
tfSang.setBounds(30, 150, 80, 30);
this.add(tfSang);
tfSu=new JTextField();
tfSu.setBounds(130, 150, 80, 30);
this.add(tfSu);
tfDan=new JTextField();
tfDan.setBounds(230, 150, 80, 30);
this.add(tfDan);
btnAdd=new JButton("추가");
btnAdd.setBounds(150, 300, 80, 30);
this.add(btnAdd);
btnDel=new JButton("삭제");
btnDel.setBounds(350, 300, 80, 30);
this.add(btnDel);
//이벤트 추가 삭제
btnAdd.addActionListener(this);
btnDel.addActionListener(this);
//테이블생성
String[] title= {"상품명","수량","단가","총금액"};
model=new DefaultTableModel(title, 0); //0은 행개수
table=new JTable(model);
JScrollPane js=new JScrollPane(table);
js.setBounds(700, 100, 500, 500);
this.add(js);
//테이블 클릭시 선택한 행번호 selectRow에 저장
table.addMouseListener(new MouseAdapter() {
@Override
public void mouseClicked(MouseEvent e) {
selectRow=table.getSelectedRow(); //행번호 저장
super.mouseClicked(e);
}
});
}
//추가,삭제 이벤트
@Override
public void actionPerformed(ActionEvent e) {
Object ob=e.getSource();
if(ob==btnAdd)
{
String sang=tfSang.getText().trim();
String su=tfSu.getText().trim();
String dan=tfDan.getText().trim();
if(sang.length()==0 || su.length()==0 || dan.length()==0)
{
JOptionPane.showMessageDialog(this, "3개값 모두 입력");
return;
}
//su랑 dan 합계..형변환
int total=0;
total=Integer.parseInt(su)*Integer.parseInt(dan);
NumberFormat nf=NumberFormat.getInstance();
Vector<String>data=new Vector<String>();
data.add(sang);
data.add(su);
data.add(dan);
data.add(nf.format(total));
//테이블에 추가
model.addRow(data);
//입력값 지워주기
tfSang.setText("");
tfSu.setText("");
tfDan.setText("");
}else if(ob==btnDel)
{
//선택 안 했을 때
if(selectRow==-1)
{
JOptionPane.showMessageDialog(this, "삭제할 행을 먼저 선택");
} else
{
model.removeRow(selectRow); //지워질 행 removeRow
//선택했을 때 removeRow를 이용해 삭제후 다시 -1로 가야 한다
selectRow=-1;
//디자인 가서 이벤트 처리해주기
}
}
}
public static void main(String[] args) {
new JTableAdd_03("JTable 추가연습");
}
}
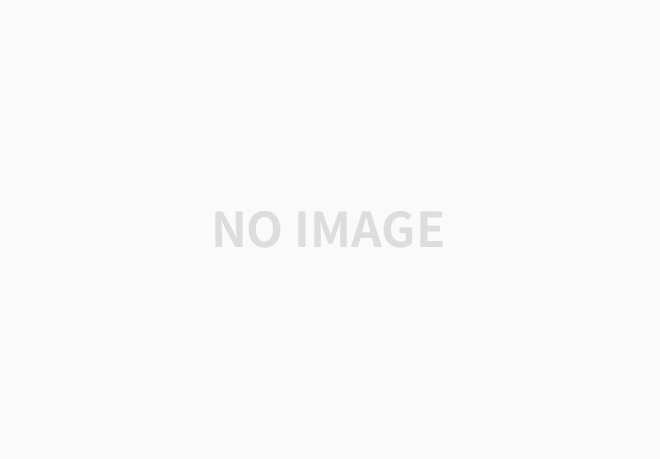
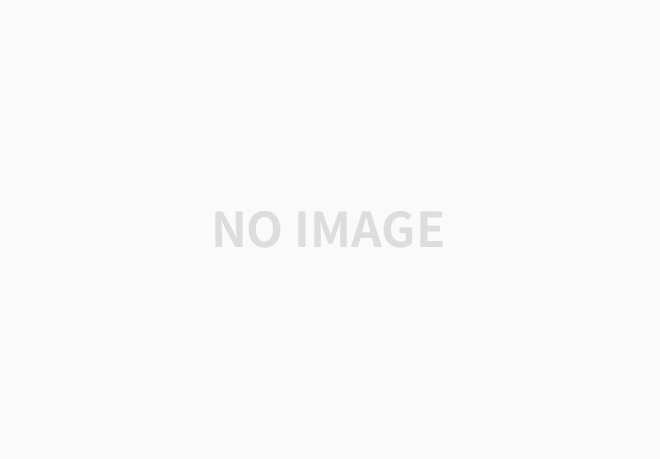
'Swing' 카테고리의 다른 글
210826_JTable+JTextArea (0) | 2021.08.26 |
---|---|
210826_JLabel+JTable+JTextArea (0) | 2021.08.26 |
210824_Swing+label+Icon+Date (0) | 2021.08.24 |
210824_Swing+Checkbox+ActionListener+ItemListener (0) | 2021.08.24 |
210824_Swing+Label+Button+Arrays+Random (0) | 2021.08.24 |